How to Use JavaScript’s .every() and .some() for Smarter Array Handling
JavaScript provides a variety of array methods that allow developers to perform common tasks in an efficient, clean, and readable manner. Among these methods are .every()
and .some()
, which offer powerful ways to evaluate arrays based on specific conditions. While these two methods are similar in concept, they serve different purposes and can be leveraged in a variety of scenarios to make your code more concise and readable.
This article delves into the details of the .every()
and .some()
methods in JavaScript, explaining their syntax, use cases, and providing examples of how and when to use them. By the end, you'll have a solid understanding of these methods and how to implement them effectively in your projects.
Understanding the Basics of .every() and .some()
Both .every()
and .some()
are array prototype methods that allow you to check if an array meets certain criteria by evaluating each element. Here's a quick breakdown of what they do:
.every()
: Tests whether all elements in an array pass the test implemented by a provided function. If every element satisfies the condition, it returnstrue
. If at least one element fails the condition, it returnsfalse
..some()
: Tests whether at least one element in an array passes the test implemented by the provided function. If any one element meets the condition, it returnstrue
. If no elements satisfy the condition, it returnsfalse
.
Both methods are commonly used for condition-based checks on arrays, such as validating form inputs, filtering data, or ensuring that certain constraints are met in collections.
1. JavaScript .every() Method
The .every()
method checks whether every element in an array passes a condition specified by a callback function. If all elements meet the condition, the method returns true
. Otherwise, it returns false
. It’s important to remember that the callback function should return a boolean value (true
or false
) for each element.
👉 Download Javascript: from ES2015 to ES2023 - eBook
Syntax
array.every(callback(element[, index[, array]])[, thisArg])
callback: A function that will be called for each element in the array. The function should return
true
if the element satisfies the condition andfalse
otherwise.element: The current element being processed in the array.
index (optional): The index of the current element being processed.
array (optional): The array that the
.every()
method was called upon.thisArg (optional): A value to use as
this
when executing the callback.
Example 1: Basic Usage of .every()
Let’s start with a simple example. Suppose we want to check if all elements in an array are greater than 10:
const numbers = [12, 14, 18, 20];
const allGreaterThanTen = numbers.every(num => num > 10);
console.log(allGreaterThanTen); // Output: true
In this case, .every()
returns true
because all numbers in the array are greater than 10.
Example 2: .every() with Mixed Results
If at least one element fails the condition, the method will return false
:
const numbers = [12, 9, 18, 20];
const allGreaterThanTen = numbers.every(num => num > 10);
console.log(allGreaterThanTen); // Output: false
Here, the second element (9
) is not greater than 10, so .every()
returns false
.
Use Cases for .every()
The .every()
method is commonly used in scenarios where you need to validate data or check whether every element of an array satisfies a particular condition. Some typical use cases include:
Form Validation: Check if all required fields in a form have been filled out or if all inputs meet validation rules.
Data Validation: Verify that all items in a data set conform to certain constraints (e.g., all numbers in an array are positive, all strings have a certain length, etc.).
Checking Access Permissions: Determine if all users in a group have a specific permission level.
2. JavaScript .some() Method
The .some()
method, on the other hand, checks whether at least one element in an array passes a condition specified by the callback function. It will return true
as soon as it finds one element that satisfies the condition. If no elements pass the test, the method returns false
.
👉 Download Javascript: from ES2015 to ES2023 - eBook
Syntax
array.some(callback(element[, index[, array]])[, thisArg])
callback: A function that will be called for each element in the array, similar to
.every()
. It should returntrue
if the element satisfies the condition andfalse
otherwise.element: The current element being processed in the array.
index (optional): The index of the current element being processed.
array (optional): The array that
.some()
was called upon.thisArg (optional): A value to use as
this
when executing the callback.
Example 1: Basic Usage of .some()
Let’s consider an example where we check if any number in the array is even:
const numbers = [3, 5, 7, 8, 11];
const hasEvenNumber = numbers.some(num => num % 2 === 0);
console.log(hasEvenNumber); // Output: true
In this case, .some()
returns true
because the number 8
is even.
Example 2: .some() with No Match
If no elements satisfy the condition, .some()
returns false
:
const numbers = [3, 5, 7, 9, 11];
const hasEvenNumber = numbers.some(num => num % 2 === 0);
console.log(hasEvenNumber); // Output: false
Here, none of the elements in the array are even, so the method returns false
.
Use Cases for .some()
The .some()
method is ideal for cases where you want to check if at least one element in an array meets a particular criterion. Common use cases include:
Checking for Errors: Determine if there is at least one error in a list of operations or data entries.
Searching for an Item: Check if any element in a shopping cart has a particular item or category.
Feature Flags: Evaluate whether at least one feature in an application is enabled or available to a user.
Differences Between .every() and .some()
While both methods are used to evaluate elements in an array, there are key differences between them:
Return Values:
.every()
returnstrue
only if all elements satisfy the condition..some()
returnstrue
if any element satisfies the condition.Short-Circuiting:
In.every()
, the iteration stops as soon as an element fails the condition because the result can no longer betrue
.
In.some()
, the iteration stops as soon as an element passes the condition since the result will definitely betrue
.Use Case:Use
.every()
when you want to ensure that all items meet a specific requirement.Use.some()
when you only need one item to meet the requirement.
Conclusion
JavaScript’s .every()
and .some()
array methods provide efficient ways to test elements in an array for specific conditions. While they seem similar at first glance, they serve distinct purposes. .every()
ensures that all elements meet the criteria, while .some()
checks if at least one element passes the test.
By understanding and applying these methods, you can write cleaner, more expressive code that is easy to maintain and debug. Whether you are performing data validation, error checking, or working with feature flags, these methods are invaluable tools in any JavaScript developer’s toolkit.
👉 Download Javascript: from ES2015 to ES2023 - eBook
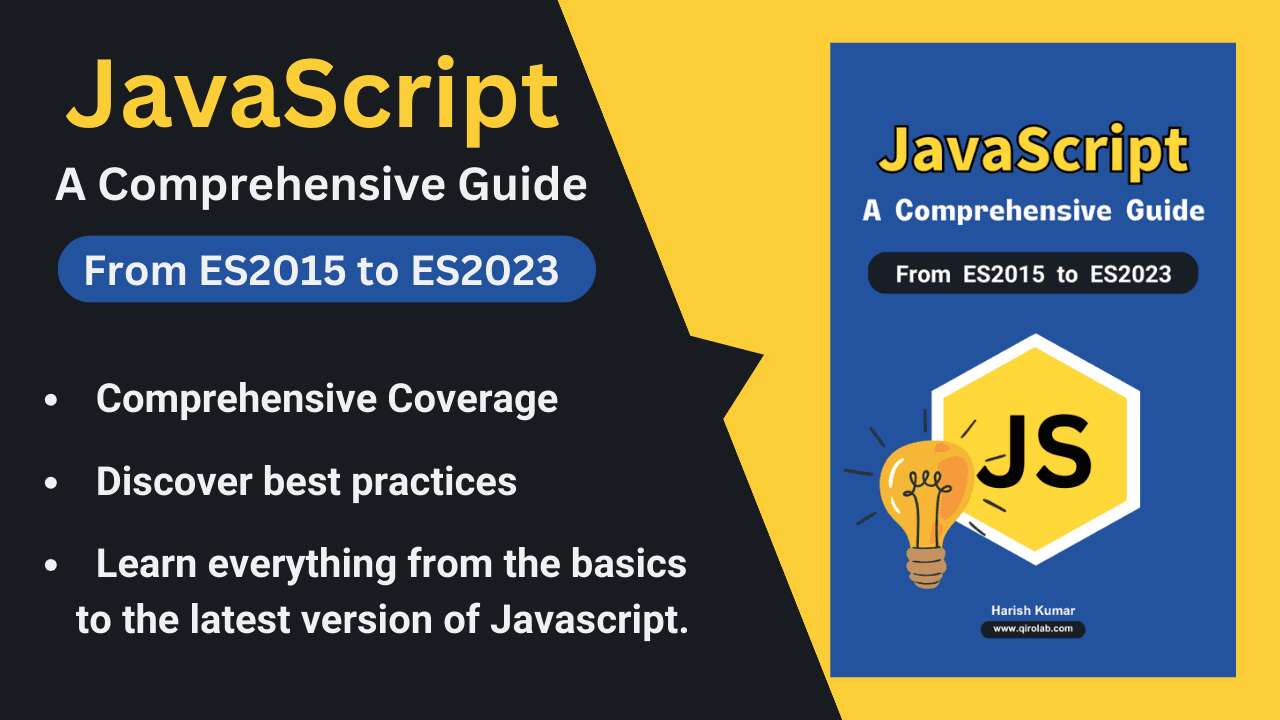
Please login or create new account to add your comment.