Exploring the Latest Features in Laravel 11.42: Date Helpers, Validation Upgrades, and More
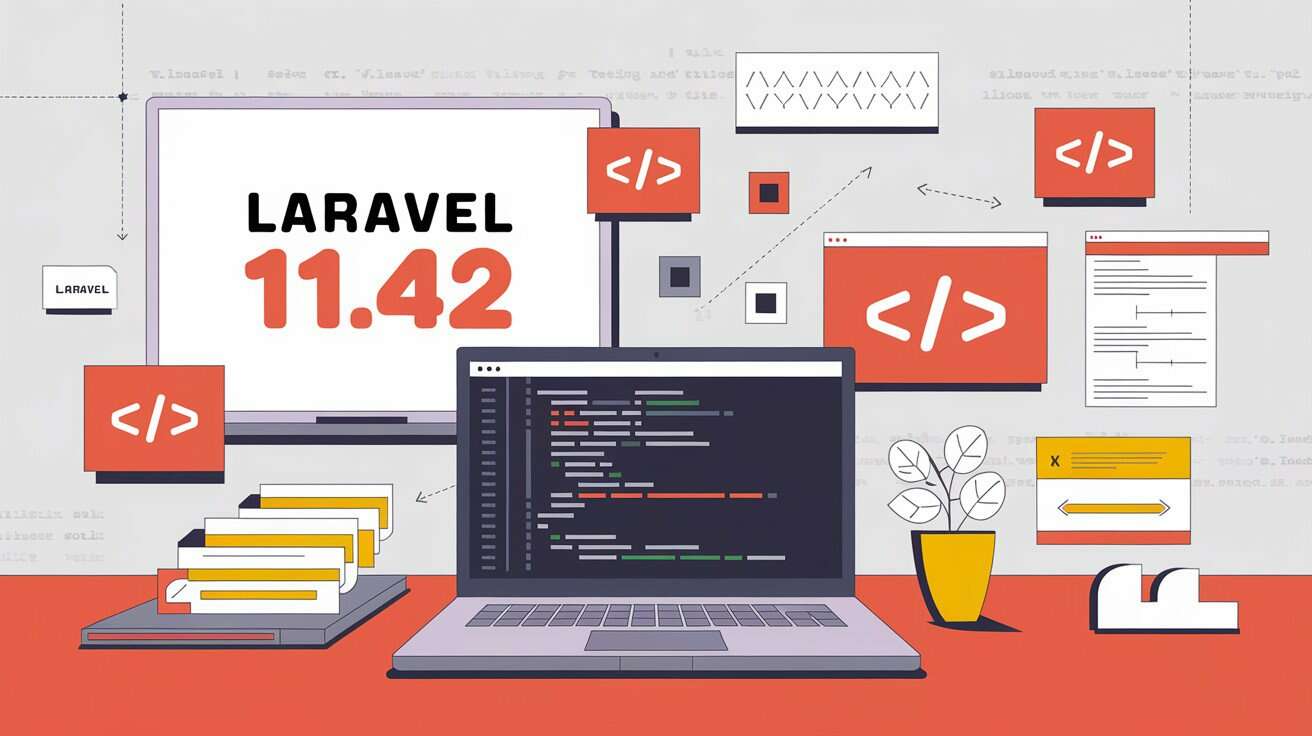
Laravel continues to evolve with its latest release, version 11.42, bringing a host of developer-friendly features to streamline workflows and enhance code expressiveness. Whether you're working with date queries, validation rules, or API testing, this update has something for everyone. Let’s dive into the highlights!
Simplifying Date Queries with Relative Date Helpers
Working with dates in database queries just got a whole lot easier. Thanks to a contribution by Jason McCreary, Laravel now includes a suite of expressive methods for querying relative dates. These helpers allow you to write cleaner, more readable code when filtering records based on dates like due_at
, created_at
, or any other timestamp columns.
For example, imagine you want to retrieve invoices that are past their due date. Previously, you might have manually calculated dates with Carbon
. Now, you can simply write:
DB::table('invoices')
->wherePast('due_at')
->get();
DB::table('invoices')
->whereFuture('due_at')
->get();
DB::table('invoices')
->whereNowOrPast('due_at')
->get();
DB::table('invoices')
->whereNowOrFuture('due_at')
->get();
DB::table('invoices')
->whereToday('due_at')
->get();
DB::table('invoices')
->whereBeforeToday('due_at')
->get();
DB::table('invoices')
->whereAfterToday('due_at')
->get();
Need flexibility? The methods also support orWhere
, orWhereNot
, and even accept an array of columns:
DB::table('invoices')
->whereToday(['due_at', 'sent_at'])
->get();
This feature is a game-changer for readability and reduces the risk of date-related bugs. For implementation details, check out Pull Request #54408.
Fluent Numeric Validation: Write Cleaner Rules
Validating numeric fields just became more elegant. Carlos Junior introduced a fluent interface for defining numeric validation rules, replacing clunky pipe-separated strings. Compare the old and new approaches:
Before:
$rules = [
'score' => 'numeric|integer|multiple_of:10|lte:some_field|max:100',
];
After:
$rules = [
'score' => [
Rule::numeric()
->integer()
->multipleOf(10)
->lessThanOrEqual('some_field')
->max(100)
],
];
This fluent syntax makes complex validation rules easier to read and maintain. No more guessing what lte
stands for—methods like lessThanOrEqual()
are self-documenting.
Checking Missing Context Keys
Laravel’s Context service, which allows sharing values across logging contexts, now includes a missing()
method. Contributed by Vincent Bergeron, this addition lets you check if a specific key is absent from the context:
Context::add('url', $request->url());
Context::addHidden('hidden_url', $request->url());
Context::missing('url'); // false
Context::missing('missing_key'); // true
// Check hidden context:
Context::missingHidden('hidden_url'); // false
This is particularly useful for ensuring sensitive data isn’t accidentally logged or for conditional logging based on context availability.
Testing Streamed JSON Responses Made Easy
Testing streamed JSON responses (useful for large datasets) used to be tricky, but Günther Debrauwer has simplified it. Now, you can use familiar JSON assertions directly on streamed content:
// In a route:
Route::get('/users', function () {
return response()->streamJson([
'data' => User::cursor(),
]);
});
// In a test:
$this->getJson('/users')
->assertJsonCount(10, 'data')
->assertJsonPath('data.*.id', $users->pluck('id')->all());
This eliminates the need to manually handle streamed data, making API testing smoother and more intuitive.
Wrapping Up
Laravel 11.42 packs powerful yet subtle improvements that reflect the framework’s commitment to developer experience. From expressive date queries to fluent validation and enhanced testing tools, these updates help you write cleaner, more maintainable code.
For a full list of changes, check out the official release notes or the original Laravel News article. Happy coding! 🚀
Please login or create new account to add your comment.