Understanding the `.reduce()` Method in JavaScript
The .reduce()
method in JavaScript is one of the most powerful array methods used for iterating over array elements and accumulating a single value from them. Whether you're summing numbers, flattening arrays, or even performing complex data transformations, reduce()
is a highly versatile and useful function in JavaScript.
In this article, we'll take a deep dive into the reduce()
method, including its syntax, common use cases, and practical examples.
👉 Download Javascript: from ES2015 to ES2023 - eBook
1. What is the .reduce() Method?
The .reduce()
method executes a reducer function (that you provide) on each element of the array, resulting in a single output value. The method is commonly used when you need to combine array elements into a single result, which can be anything from a number to an object or even another array.
In short, .reduce()
helps you "reduce" the array into a singular value by processing each element iteratively.
2. Syntax of .reduce()
array.reduce(callback(accumulator, currentValue, currentIndex, array), initialValue)
3. Parameters of .reduce()
Callback Function: The function to be executed on each element of the array.
accumulator
: The accumulator accumulates the return values of the callback function. It's essentially the "running total" or result so far.currentValue
: The current element being processed.currentIndex
(Optional): The index of the current element.array
(Optional): The array on which the.reduce()
method is called.initialValue (Optional): The initial value of the accumulator. If omitted, the first element of the array is used as the initial value, and the callback starts from the second element.
4. How Does .reduce() Work?
The reduce()
method works by iterating over each item in an array and applying the callback function to it, with the accumulator storing the result. It processes the elements from left to right in the array and returns the accumulated result at the end.
Example:
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
}, 0);
console.log(sum); // Output: 10
In this example:
The
reduce()
method starts with an initial value of0
(theinitialValue
).It adds each element (
currentValue
) to theaccumulator
.By the end, the
accumulator
contains the sum of all elements, which is10
.
5. Common Use Cases
a. Summing Numbers
One of the most common use cases for reduce()
is summing numbers in an array.
Example:
const numbers = [10, 20, 30, 40];
const total = numbers.reduce((sum, num) => sum + num, 0);
console.log(total); // Output: 100
b. Flattening Arrays
You can use reduce()
to flatten a multidimensional array.
Example:
const nestedArray = [[1, 2], [3, 4], [5, 6]];
const flattened = nestedArray.reduce((acc, curr) => acc.concat(curr), []);
console.log(flattened); // Output: [1, 2, 3, 4, 5, 6]
c. Counting Occurrences
If you need to count the occurrence of specific values in an array, reduce()
is an excellent tool for the job.
Example:
const fruits = ['apple', 'banana', 'orange', 'apple', 'banana', 'apple'];
const count = fruits.reduce((acc, fruit) => {
acc[fruit] = (acc[fruit] || 0) + 1;
return acc;
}, {});
console.log(count);
// Output: { apple: 3, banana: 2, orange: 1 }
d. Grouping Data
You can also group items in an array by a certain property using reduce()
.
Example:
const people = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 25 }
];
const groupedByAge = people.reduce((acc, person) => {
const ageGroup = person.age;
if (!acc[ageGroup]) {
acc[ageGroup] = [];
}
acc[ageGroup].push(person.name);
return acc;
}, {});
console.log(groupedByAge);
// Output: { 25: ['Alice', 'Charlie'], 30: ['Bob'] }
6. Important Considerations
Initial Value: Always provide an initial value unless you have a very good reason not to. If the array is empty and no initial value is provided,
reduce()
will throw a TypeError.Immutability: Avoid mutating the accumulator or currentValue directly. Instead, return new values in every iteration to maintain immutability.
Complexity: Using
reduce()
can sometimes make your code harder to read. Make sure it’s appropriate for the task and doesn't overcomplicate things.
7. Conclusion
The .reduce()
method is an extremely powerful and flexible tool for working with arrays in JavaScript. Whether you’re summing up numbers, flattening nested arrays, counting occurrences, or grouping data, reduce()
provides an elegant solution. However, it’s crucial to use it wisely, keeping readability and performance in mind.
By mastering reduce()
, you'll have a deeper understanding of how to process data and perform advanced transformations in JavaScript arrays.
👉 Download Javascript: from ES2015 to ES2023 - eBook
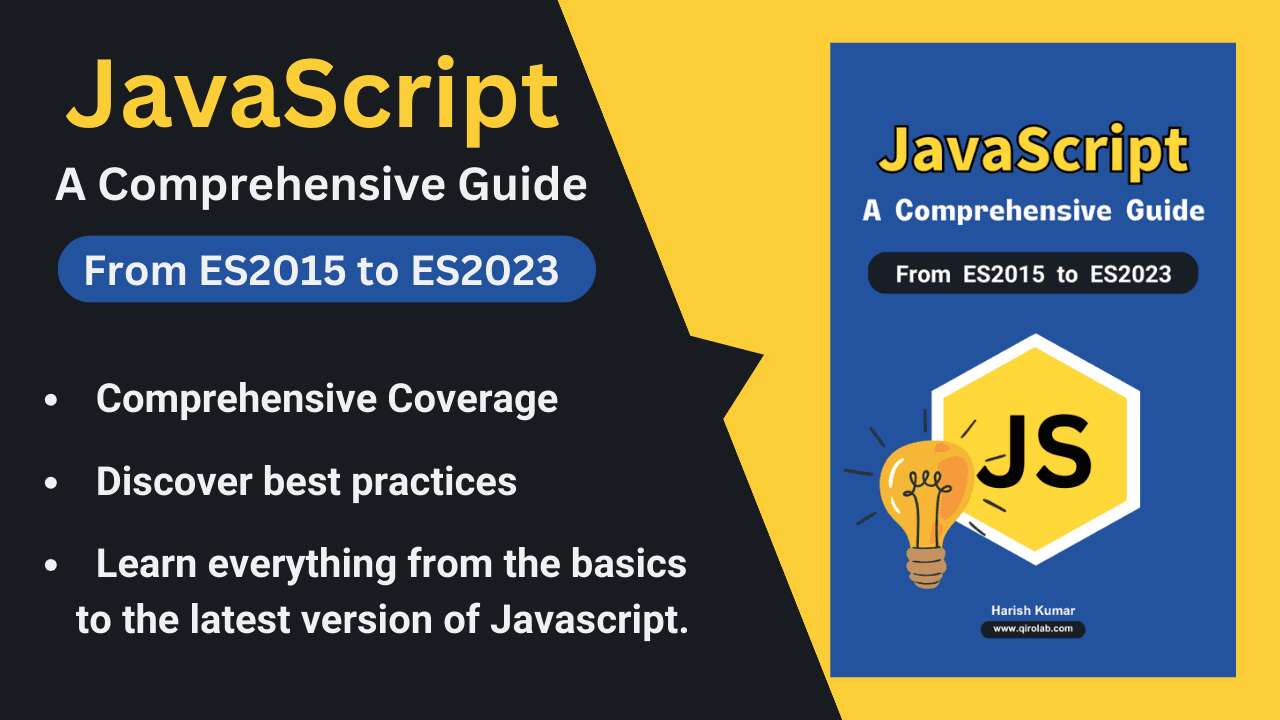
Please login or create new account to add your comment.